How to call a background task with hosted service from .NET core web API?
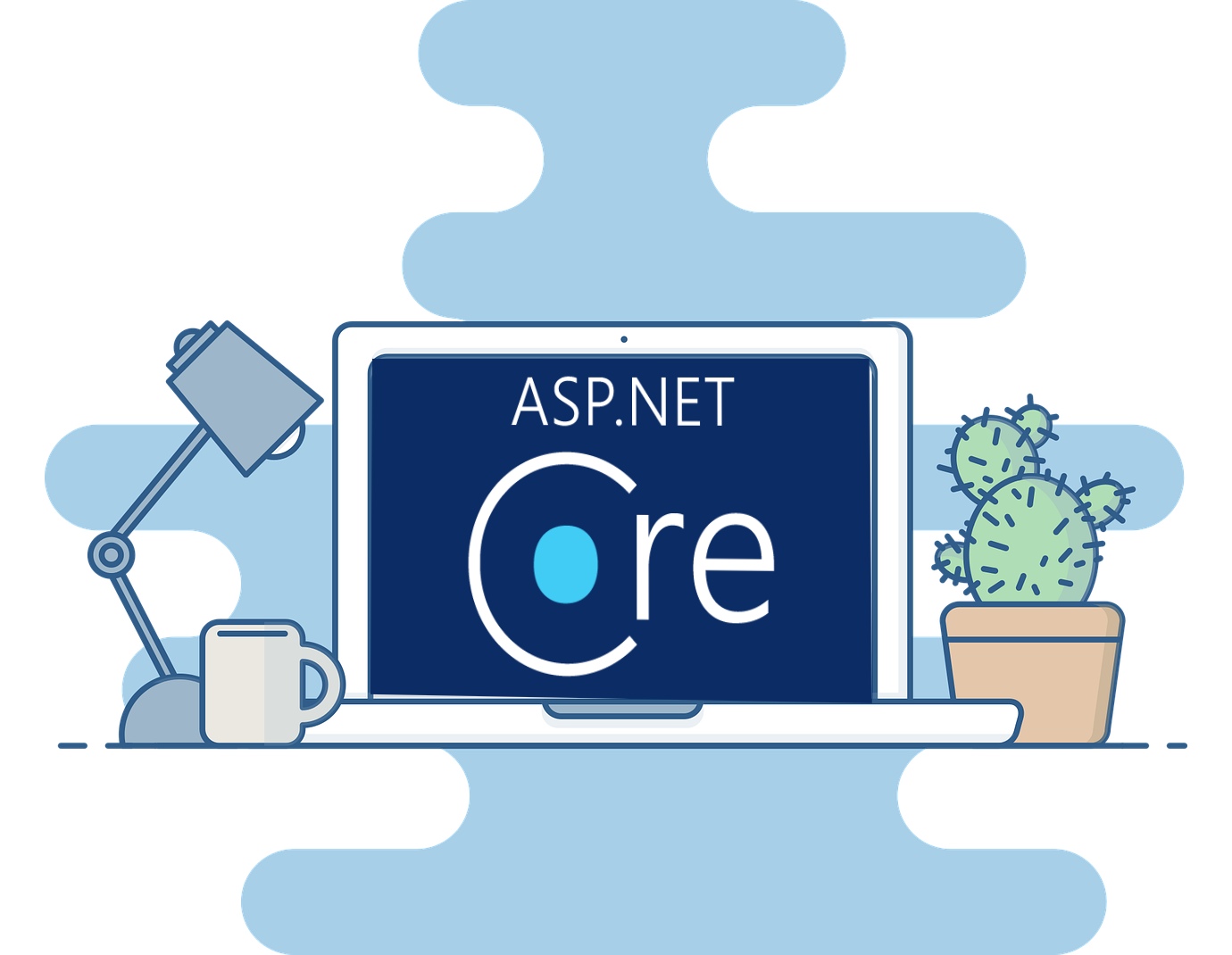
Over the years Microsoft .Net has been among the top choices for project development as it has several updated framework versions like Asp.Net, WPF, Asp.Net Core, etc. Asp.Net Core is on the hype for making robust development because of cross-platform compatibility. Asp.Net core includes class libraries and strong security features that are indispensable for any application these days.
Today, we will be going to walk you through the ASP.NET Core web applications on how to perform background tasks using the infrastructure and API provided by the ASP.NET Core framework.
There are many scenarios wherever you want to run a task continuously in the background. Oftentimes, we create our own interfaces and classes to achieve this functionality and somehow wire them up in the Startup class.
In this blog post, we will cover two main ways to run background tasks in the ASP.NET core web application. Each of the ways are provided out of the box by the ASP.Net Core Framework.
We do not need an external NuGet package to execute these background tasks.
Application for background tasks
To begin this example, we will first create a new ASP.NET Core Web API application.
First, to do that, we will open Visual Studio 2019. Once Visual Studio opens, we will select File -> New -> Project menu. This new project will open the Create Project popup window.
Second, create a new project in the popup window, we will select the ASP.NET Core web application from the project template and click the Next button.
Thirdly, on the next page, we will provide the name of the application as BackgroundTask.Demo and click on the Create button.
Finally, on the final page, we will select the API template option. And we will keep the other values as default (ASP.Net Core 3.1) and click the Create button.
Two ways to execute background tasks
As we said earlier, we can perform background actions in the ASP.NET core using two different constructs provided by the ASP.NET Core framework.
They are as follows:
- First, we can implement the IHostedService interface
- Second, we can get the BackgroundService from the abstract base class
Background tasks using IHostedService
Now that the project is ready, it’s time to create our first background tasks. To create a continuous background task, let us consider that we have a printing process. It will print an additional integer number.
To achieve this, we will create a new class BackgroundPrinter. This class can implement the IHostedService interface.
The IHostedService interface offers two methods, StartAsynch and StopAsynch. The StartAsync method is wherever the task should begin. We should apply logic when there’s a StopAsync method where the task is stopped.
Some important points to remember about the StartAsync method:
- First, the Startup class configuration method is called the StartAsync method by the framework before.
- Secondly, the StartAsync method is termed before the server starts.
Finally, if the class that implements the IHostedService uses an unmanaged object, the class should implement an IDisposable interface to lose the unmanaged object.
IHostedService implementation with a Timer object
For the first version of the code, we will apply a Timer within the BackgroundPrinter class. And at the timer interval, we will print the enhanced number.
First, we will declare the timer object and the integer variable number in the class.
Second, inside StartAsync We will create a new example of a timer.
Third, for the timer representative, we will define an anonymous function, where we will multiply and print an integer using ILogger defined as a class-level variable.
Fourth, we will set the timer to run at 6-second intervals.
Finally, we will apply the IDisposable interface to call the timer object dispose method and implement the disposal method.
using Microsoft.Extensions.Hosting;
using Microsoft.Extensions.Logging;
using System;
using System.Threading;
using System.Threading.Tasks;
namespace BackgroundTask.Demo
{
public class BackgroundPrinter : IHostedService, IDisposable
{
private readonly ILogger<BackgroundPrinter> logger;
private Timer timer;
private int no;
public BackgroundPrinter(ILogger<BackgroundPrinter> logger,
IWorker worker)
{
this.logger = logger;
}
public void Dispose()
{
timer?.Dispose();
}
public Task StartAsync(CancellationToken cancellationToken)
{
timer = new Timer(o => {
Interlocked.Increment(ref no);
logger.LogInformation($”Print the worker no{no}”);
},
null,
TimeSpan.Zero,
TimeSpan.FromSeconds(6));
return Task.CompletedTask;
}
public Task StopAsync(CancellationToken cancellationToken)
{
return Task.CompletedTask;
}
}
}
Configuring the BackgroundPrinter class
Once the BackgroundPrinter class is ready, we will now configure it on a dependent injection container using the AddHostedService extension method on the IServiceCollection interface.
Now, we can do that in Startup class or program class. We prefer to do that in program class, just for the obvious division.
So, we will update the program class to achieve this. In the CreatHostBuilder method, we will use the ConfigureServices extension method on the IHostBuilder interface to do this.
using Microsoft.AspNetCore.Hosting;
using Microsoft.Extensions.DependencyInjection;
using Microsoft.Extensions.Hosting;
namespace BackgroundTask.Demo
{
public class Test
{
public static void Main(string[] args)
{
CreateHostBuilder(args).Build().Run();
}
public static IHostBuilder CreateHostBuilder(string[] args) =>
Host.CreateDefaultBuilder(args)
.ConfigureWebHostDefaults(webBuilder =>
{
webBuilder.UseStartup<Startup>();
}).ConfigureServices(services =>
services.AddHostedService<BackgroundPrinter>());
}
}
Background tasks using BackgroundService
Using the BackgroundService Abstract Base class is another way to perform background tasks. Implementing a BackgroundService is relatively easy compared to an IHostedService. But at the same time, you have less control over how to start and stop work.
To demonstrate how the BackgroundService Abstract Base Class works, we will create a new class DerivedBackgroundPrinter. This new class will run from the BackgroundService class.
And this time, we have just use the IWorker interface for business logic. So, we will create a constructor dependency on the IWorker interface. Since the iWorker interface dependency is already configured in the injection container, it will automatically pass with this class.
The BackgroundService Abstract Base Class provides one abstract method ExecuteAsync. we are going to have our implementation call the DoWork call of the Iworker interface within this ExecuteAsync method.
using Microsoft.Extensions.Hosting;
using System.Threading;
using System.Threading.Tasks;
namespace BackgroundTask.Demo
{
public class DerivedBackgroundPrinter : BackgroundService
{
private readonly IWorker worker;
public DerivedBackgroundPrinter(IWorker worker)
{
this.worker = worker;
}
protected override async Task ExecuteAsync(CancellationToken stoppingToken)
{
await worker.DoWork(stoppingToken);
}
}
}
Once this class is ready, we will update the program class to use the DerivedBackgroundPrinter class instead of the BackgroundPrinter as the background task runner.
So, within the CreateHostBuilder method of the Program class, we will replace the BackgroundPrinter with a DerivedBackgroundPrinter for the AddHostedService generic extension method call.
using Microsoft.AspNetCore.Hosting;
using Microsoft.Extensions.DependencyInjection;
using Microsoft.Extensions.Hosting;
namespace BackgroundTask.Demo
{
public class Test
{
public static void Main(string[] args)
{
CreateHostBuilder(args).Build().Run();
}
public static IHostBuilder CreateHostBuilder(string[] args) =>
Host.CreateDefaultBuilder(args)
.ConfigureWebHostDefaults(webBuilder =>
{
webBuilder.UseStartup<Startup>();
}).ConfigureServices(services =>
services.AddHostedService<DerivedBackgroundPrinter>());
}
}
Conclusion
In this article, we have learned about how to call a background task with hosted service from .NET Core Web API. As you can see, it is extremely easy to create background tasks using the IHostedService interface as well as the background Service Abstract Base Class via ASP.NET Core. The most effective is that there’s no external NuGet package dependence.
Author Bio: Vinod Satapara – Technical Director, iFour Technolab Pvt. Ltd.
Technocrat and entrepreneur with years of experience building large scale enterprise web, cloud and mobile applications using latest technologies like ASP.NET, CORE, .NET MVC, Angular and Blockchain. Keen interest in addressing business problems using latest technologies and help organization to achieve goals.
Hire Asp.Net Core developers from iFour Technolab to get your requirements done.