Custom Action Filters in ASP.NET MVC
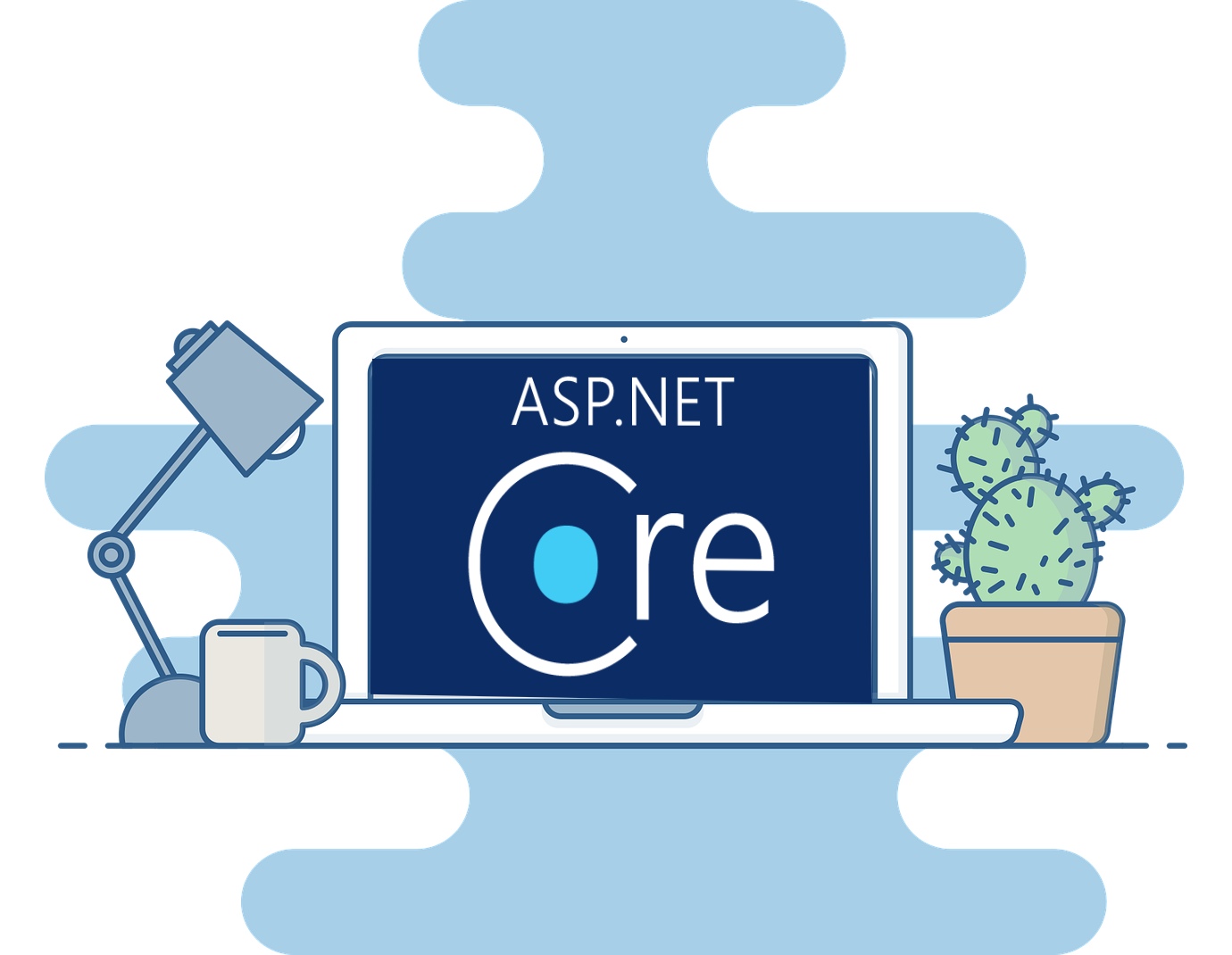
What is ASP.NET Filter?
In MVC, the user’s request is routed to a specific controller and action method. Although, there might be situations where one wants to apply logic prior to and after any action method execution. Different types of ASP.NET Filters are applied to use this purpose.
ASP.NET Filter is a custom class that is used to write custom logic for execution prior and/or after an action method is executed. It can be applied to any action or controller.
There are mainly four types of filters:
- Authorization Filter
- Action Filter
- Result Filter
- Exception Filter
Each type is executed at a different stage in the pipeline and thus has its own set of intended cases. Choose what kind of filter to create based on the task you need it to perform, and wherein request pipeline it executes.
-
What is Action Filter?
Action filter’s execution happens before and after an action method executes. Action filter attributes can be applied to a specific action and/or to a controller. When an action filter is applied to a controller, it is applied to all the action methods.
The OutputCache is a system action filter attribute that can be used to an action method for which we want to cache the output.
For example, the output of this action method will be cached for 50 seconds.
[OutputCache(Duration=50)]
public ActionResult Index()
{
return View();
}
- Prerequisites
Microsoft Visual Studio Express for Web or superior
- Setup
For ease, much of the code you will be managing along this is available as Visual Studio code snippets. To install the code snippets run .\Source\Setup\CodeSnippets.vsi file.
- How to create a custom Action Filter?
There are two ways to create a custom action filter:
- By Implementing the IActionFilter interface and the FilterAttribute class
- By deriving the ActionFilterAttribute abstract class
A custom action filter class performs inheritation from the FilterAttribute class. Based on one’s requirements you may need to perform the following steps:
- Decide whether your custom action filter needs to have any order in the execution chain.
- Depending on the requirements, implement IAuthorizationFilter or IActionFilter or IResultFilter or IExceptionFilter.
- If you are doing implementation of IAuthorizationFilter interface then write implementation for OnAuthorization() method.
- If you are doing implementation of IActionFilter interface then write implementation for OnActionExecuting() and OnActionExecuted() methods.
- If you are doing implementation of IResultFilter interface then write implementation for OnResultExecuting() and OnResultExecuted() methods.
- If you are doing implementation of from IExceptionFilter interface then write implementation for OnException() method.
- Decoration of action methods with one or more action filters.
- Steps to create a custom Action Filter:
- Create a class
- Inherit it from ActionFilterAttribute class
- Override at least one of these methods:
- OnActionExecuting
- OnActionExecuted
- OnResultExecuting
- OnResultExecuted
Example:
using System;
using System.Diagnostics;
using System.Web.Mvc;
namespace WebApplication1
{
public class MyFirstCustomFilter : ActionFilterAttribute
{
public override void OnResultExecuting(ResultExecutingContext filterContext)
{
filterContext.Controller.ViewBag.GreetMesssage = “Hello World”;
base.OnResultExecuting(filterContext);
}
public override void OnActionExecuting(ActionExecutingContext filterContext)
{
var controllerName = filterContext.RouteData.Values[“controller”];
var actionName = filterContext.RouteData.Values[“action”];
var message = String.Format(“{0} controller:{1} action:{2}”, “onexecuting”, controllerName, actionName);
Debug.WriteLine(message, “Action Filter Log”);
base.OnActionExecuting(filterContext);
}
}
In this program, we are setting ViewBag property for the controllers being executed. The ViewBag property must be set prior to a controller action result is executed since we are overriding the OnResultExecuting method. Also, it is overriding OnActionExecuting to log the information about the controller’s action method.
If you want to increase the security of a certain section of your website, you can place an [Authorize] attribute on an ActionResult method in your controller. If someone visits your website and they try to hit that page and they aren’t authorized, they are sent to the login page (Of course, you need to modify your web.config to point to the login page for this to work properly).
public class RequiresAuthenticationAttribute : ActionFilterAttribute
{
public override void OnActionExecuting(ActionExecutingContext context)
{
var httpContext = context.HttpContext;
if (!httpContext.User.Identity.IsAuthenticated)
{
if (httpContext.Request.Url != null)
{
var redirectOnSuccess = httpContext.Request.Url.AbsolutePath;
var urlHelper = new UrlHelper(context.RequestContext);
var url = urlHelper.LoginUrl(redirectOnSuccess);
httpContext.Response.Redirect(url, true);
}
}
base.OnActionExecuting(context);
}
}
- Possible levels of Action Filters
- As Global Filter
A filter can be added to the global filter in App_Start\FilterConfig. After applying this one can use this for all the controllers in the Application.
public class FilterConfig { public static void RegisterGlobalFilters(GlobalFilterCollection filters) { filters.Add(new HandleErrorAttribute()); filters.Add(new MyFirstCustomFilter()); } }
- At Controller Level
To apply the filter at controller level, we should apply it as an attribute to a controller. At the time of applying to controller level, action will be available to all controllers.
[MyFirstCustomFilter] public class HomeController : Controller { public ActionResult Index() { return View(); } }
- At Action Level
To apply the filter at action level, we should apply it as an attribute of the action.
[MyFirstCustomFilter] public ActionResult Contact() { ViewBag.Message = “Your contact page.”; return View(); }
- Output Cache Example:
//StoreCollector.cs
using System;
using System.Collections.Generic;
using System.Linq;
using System.Web;
using System.Web.Mvc;
namespace MvcApplicationDemo.Controllers
{
public class StoreController : Controller
{
[OutputCache(Duration =10)]
public ActionResult Index()
{
return View();
}
}
}
//index.cshtml
<h4>
@{
Response.Write( DateTime.Now.ToString(“T”));
}
</h4>
OUTPUT:
- Authorization Example:
//StoreCollector.cs
using System;
using System.Collections.Generic;
using System.Linq;
using System.Web;
using System.Web.Mvc;
namespace MvcApplicationDemo.Controllers
{
public class MusicStoreController : Controller
{
[Authorize]
public ActionResult Index()
{
return View();
}
}
}
OUTPUT:
Conclusion
In ASP.NET MVC applications, the code is written in the action methods, you may want some extra prior or post processing to be carried out. ASP.NET MVC allows you to do this with the usage of Action Filters. In other words, an action filter is a class that does inheritation from the FilterAttribute base class in the library. Depending on one’s need one can implement IAuthorizationFilter, IActionFilter, IResultFilter or IExceptionFilter interfaces to make the filter an authorization filter, action filter, result filter, or exception filter respectively. These interfaces decide the procedure in which the action filters are executed.
Author Bio: Vinod Satapara – Technical Director, iFour Technolab Pvt. Ltd.
Technocrat and entrepreneur with years of experience building large scale enterprise web, cloud and mobile applications using latest technologies like ASP.NET, CORE, .NET MVC, Angular and Blockchain. Keen interest in addressing business problems using latest technologies and help organization to achieve goals.
Hire Asp.Net developers from iFour Technolab to get your requirements done.
Image:
LinkedIn: https://www.linkedin.com/in/vinodsatapara/